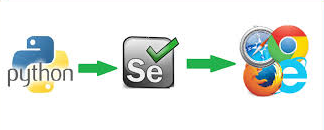
Introduction
Web scraping is an essential technique for extracting data from websites, used in various industries for research, data analysis, and automation. While Beautiful Soup and Scrapy are excellent tools for traditional HTML scraping, Selenium is a powerful tool designed for scraping dynamically loaded web pages that rely on JavaScript. This guide provides a step-by-step, SEO-optimized approach to using Selenium for web scraping.
Why Choose Selenium Over Scrapy and Beautiful Soup?
Selenium is an automation framework primarily used for web testing but is also an excellent tool for scraping JavaScript-heavy websites. Some key benefits include:
- Handles JavaScript Rendering: Can interact with elements that load dynamically.
- Automates Browser Actions: Simulates user behavior like clicks and scrolling.
- Supports Multiple Browsers: Works with Chrome, Firefox, and Edge.
- Takes Screenshots: Useful for verifying scraped content.
Prerequisites
Before starting, install the necessary dependencies:
- Python 3.8+
- Selenium library
- WebDriver for the browser (ChromeDriver, GeckoDriver, etc.)
To install Selenium, run:
pip install selenium
Download the appropriate WebDriver from the official browser site and ensure it is in your system’s PATH.
Step 1: Setting Up Selenium for Web Scraping
Start by importing the necessary modules and setting up a WebDriver:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
# Set up the WebDriver
driver = webdriver.Chrome()
driver.get("https://example.com")
Step 2: Locating and Extracting Data
Selenium provides multiple ways to locate elements using find_element()
and find_elements()
methods:
title = driver.find_element(By.TAG_NAME, "h1").text
print("Page Title:", title)
all_links = driver.find_elements(By.TAG_NAME, "a")
for link in all_links:
print(link.get_attribute("href"))
Step 3: Interacting with Web Elements
Selenium allows interaction with web pages, including clicking buttons and filling forms:
search_box = driver.find_element(By.NAME, "q")
search_box.send_keys("Web scraping with Selenium")
search_box.send_keys(Keys.RETURN)
time.sleep(3)
Step 4: Handling Infinite Scrolling
For websites with infinite scrolling, use JavaScript execution:
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
time.sleep(2)
new_height = driver.execute_script("return document.body.scrollHeight")
if new_height == last_height:
break
last_height = new_height
Step 5: Taking Screenshots
To save a screenshot of the page:
driver.save_screenshot("screenshot.png")
Step 6: Closing the WebDriver
Always close the WebDriver after scraping:
driver.quit()
Best Practices for Selenium Web Scraping
- Use Headless Mode: Reduces overhead by running without a UI.
- Implement Delays: Avoid getting blocked by adding sleep intervals.
- Rotate User Agents: Mimic real user behavior.
- Respect
robots.txt
: Adhere to website rules before scraping.
To run Chrome in headless mode:
options = webdriver.ChromeOptions()
options.add_argument("--headless")
driver = webdriver.Chrome(options=options)
Conclusion
Selenium is an excellent tool for scraping dynamic content that relies on JavaScript. By following this guide, you can extract valuable data efficiently while respecting ethical scraping guidelines.
For large-scale scraping, consider integrating Selenium with Scrapy or an API-based approach to enhance efficiency.